Going realtime with Node.js, Express and socket.io
A how to tutorial on creating a simple realtime counter in Node.js
Counting is fun! ¶
This is a walkthrough on how to create a simple realtime counter of visitors to a page using Node.js, socket.io and express. This example is very simple but could easily be extended to a range of applications.
Just show me the app ¶
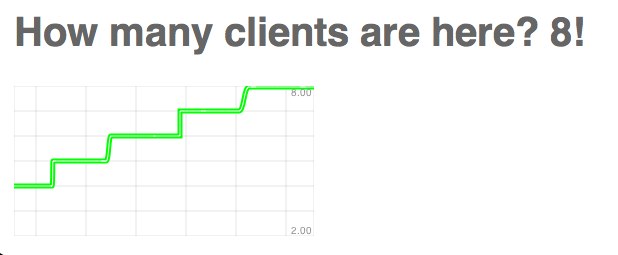
Pulling the pieces together ¶
Express is a great web framework for creating sites in Node.js. I’ve written before about creating a basic site. This time we are going to use a couple more libraries to add realtime communication to the application.
socket.io is an amazing library that takes the pain out of websockets and provides a reliable fallback depending on browser capabilites. Here’s a simple example from the socket.io site.
var io = require("socket.io").listen(80);
io.sockets.on("connection", function (socket) {
socket.emit("news", { hello: "world" });
socket.on("my other event", function (data) {
console.log(data);
});
});
This sets up the server side. Then you just need to add some client-side JavaScript and you are ready to go
<script src="/socket.io/socket.io.js"></script>
<script>
var socket = io.connect("http://localhost");
socket.on("news", function (data) {
console.log(data);
socket.emit("my other event", { my: "data" });
});
</script>
I’ve used this setup to push the number of connected clients out to the browser and a simple counter to increment and decrement the number of connected clients on the relevant events. I’ve had some issues with different browsers on hosting environments. For now the demo works with Safari and Chrome.
Smoothie Charts is a great library that will draw graphs in JavaScript. It is very customisable, and lightweight and is specifically designed for live, streaming data. It uses canvas to draw the graphs and the tutorial shows just how easy it is to create a realtime charts.
Bringing these libraries together gives us live, realtime graphs. There’s no persistence here but if we wanted to we could add something like Redis into the mix to persist the maximum number of connections.
You can browse the source and see how the libraries are used on Github. Fork it!
Potentials uses ¶
We could use this kind of setup to show realtime stats on server resources of the kind provided by Munin, to create realtime statistics for a page, or to stream live data from a third party API to a page. I’m excited about what people are going to make with these libraries!
Tags
Can you help make this article better? You can edit it here and send me a pull request.
See Also
-
Creating a basic site with Node.js and Express
A walkthrough on how to create and deploy a basic site with Node.js and the Express framework. Examples of generating an express site, how to use templating and styles, creating basic routes and deploying the app to the Internet. -
Cloud Foundry - a Ruby and Node.js developer's perspective
This week VMware announced Cloud Foundry, their open source PaaS offering. Here's my take. -
Setting up Node.js and npm on Mac OSX
Node.js is gaining a lot of speed and is an exciting new development framework. Here's a quick overview of how to get Node.js working on OSX along with npm, the package manager for node.